How to Work with TCP Sockets in Python
A network/internet socket is an end-point of interposing communication across a computer network. In Python, the library has a module called socket which offers a low-level networking interface, it’s common across various programming languages since it uses OS-level system calls. When you create a socket, use a function called a socket. It accepts family, type, and prototype arguments. Creating a TCP-socket, for the family, you must use a socket.AF_INET or socket.AF_INET6 and for the type you must use socket.SOCK_STREAM.
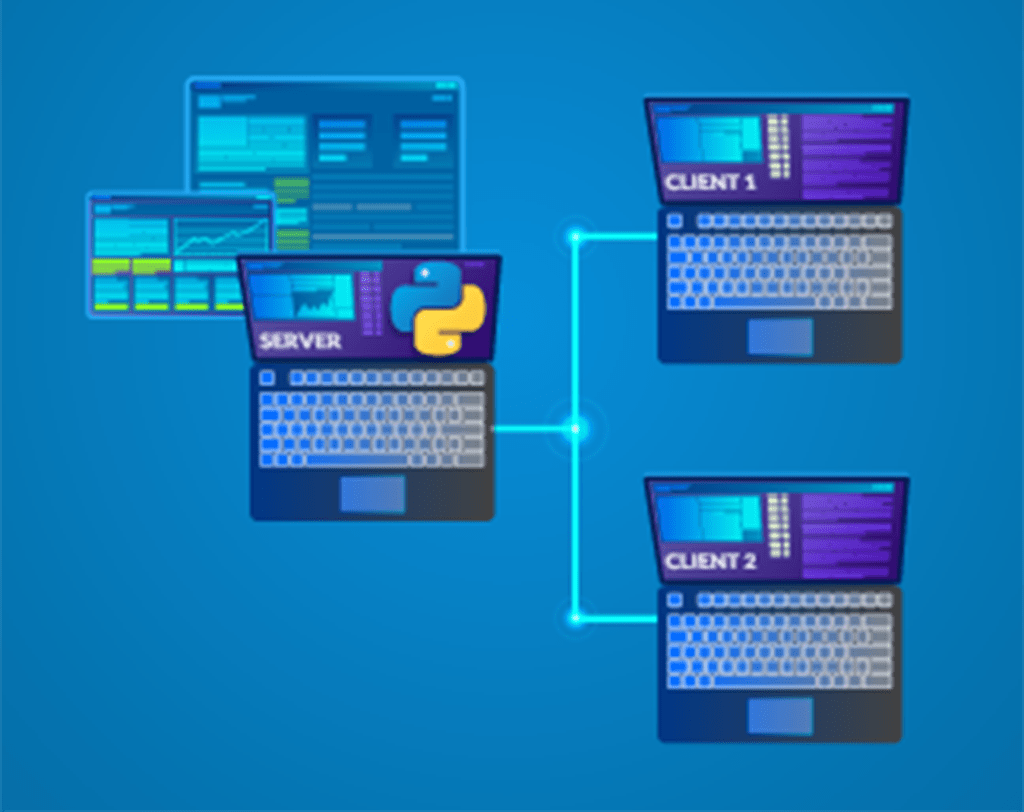
Example for Python socket
import socket
s=socket.socket(socket.AF_INET, socket.SOCK_STREAM)
Main methods to create Python socket objects
- bind() – specific for server sockets.
- listen() – specific for server sockets.
- accept() – specific for server sockets.
- connect() – client sockets.
- send() – both server and client sockets.
- recv() – both server and client sockets.
For instance, echo server from documentation
import socket
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
s.bind((‘localhost’, 50000))
s.listen(1)
conn, addr = s.accept()
while 1:
data = conn.recv(1024)
if not data:
break
conn.sendall(data)
conn.close()
To create a server socket, bind it to localhost and 50000 port, and start listening for incoming connections. To accept an incoming connection call as accept(), this method will block until a new client connects. When it’s happening, will create a new socket and returns it together with the client’s address. Then, an infinite loop reads data from the socket in batches of 1 KB using method recv() till it returns a blank string. Next, it sends all incoming data back using a method called sendall() which inside repeatedly calls send(). Next, to that, it just closes the client’s connection.
A client-side code looks simply
import socket
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
s.connect((‘localhost’, 50000))
s.sendall(‘Hello, world’)
data = s.recv(1024)
s.close()
print ‘Received’, repr(data)
Here instead of bind() and listen() methods it calls only the connect() method and directly sends data to the server. Then it receives 1 KB back, closes the socket then writes the received data. Each socket method is blocking. For instance, when it reads from a socket or prints to it the program can’t do anything. The possible solution is to delegate working with clients to separate process/threads, creating any process and swap contexts between them is not a cheap operation. To solve this issue, there is a so-called asynchronous way of working with sockets. The main idea is to maintain the socket’s state to an OS and check the program when there is something to read from the socket or when it is ready for writing.
There are a group of interfaces for various operating systems:
- poll, epoll (Linux).
- kqueue, kevent (BSD).
- select (cross-platform).
To create a server using Python select
import select, socket, sys, Queue
server = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
server.setblocking(0)
server.bind((‘localhost’, 50000))
server.listen(5)
inputs = [server]
outputs = []
message_queues = {}
while inputs:
readable, writable, exceptional = select.select(
inputs, outputs, inputs)
for s in readable:
if s is server:
connection, client_address = s.accept()
connection.setblocking(0)
inputs.append(connection)
message_queues[connection] = Queue.Queue()
else:
data = s.recv(1024)
if data:
message_queues[s].put(data)
if s not in outputs:
outputs.append(s)
else:
if s in outputs:
outputs.remove(s)
inputs.remove(s)
s.close()
del message_queues[s]
for s in writable:
try:
next_msg = message_queues[s].get_nowait()
except Queue.Empty:
outputs.remove(s)
else:
s.send(next_msg)
for s in exceptional:
inputs.remove(s)
if s in outputs:
outputs.remove(s)
s.close()
del message_queues[s]
There is much more code than in the blocking Echo server. That is principal ’cause we need to maintain a set of queues for different lists of sockets, i.e. writing, reading, and a separate list for erroneous sockets.
Creating a server socket looks the same except for within a line: server.setblocking(0). This is done to make the socket non-blocking. Now, severs are more advanced they can serve more than one client.
The most important point in selecting sockets
readable, writable, exceptional = select.select(
inputs, outputs, inputs)
Now we call select. select to ask the OS to check given sockets whether they are ready to write, read, or if there is some exception individually. That is why it passes 3 lists of sockets to specify which socket is expected to be printable, readable, and which should be checked for mistakes. This call will block the program or else a timeout argument is passed until some of the passed sockets are ready. At this time, the call will return 3 lists with sockets for particular operations.
Then it consecutively iterates over those lists and, if there are sockets in them, it performs a corresponding process. When there is the server socket in inputs. So, it calls accept (), adds a returned socket to inputs, and adding a Queue for incoming conversation which will be sent back. This happens in a repeated manner if new clients have arrived in added to the queue list.
For printable sockets, it gets pending messages and writes them to the socket. If there are any mistakes in the socket, it removes the socket from the lists. This is how sockets work at a lower-level and in most cases, there is no need to implement the logic at such a low level.